Character Movement
This is where I see a few artists go running off, please bear with me as it wont be that painful! To get our character to do some thing we need to run a script on it. Earlier in the tutorial we added a Micro Script action to the entity. This script is unique to this object and as such, you can not damage any script library file. The worst that can happen is that you break the script, which in this case will be a very easy fix. I promise!
1) Open the Script
Open this characters Micro Script by first selecting the character and RMB > Lua - [Edit] Micro Script
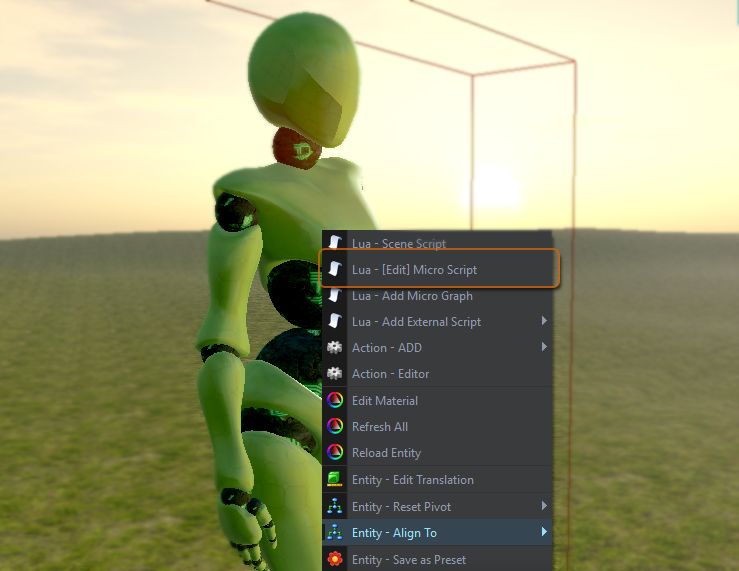
2) Script Editor
Fear not, for a lot of mechanics tutorials you can just copy and paste code directly into the script editor and save. This will be generally all you need to do unless you are feeling a bit more adventurous or of course you are a scripter. So you should have the script editor open now and looking similar to the following image.
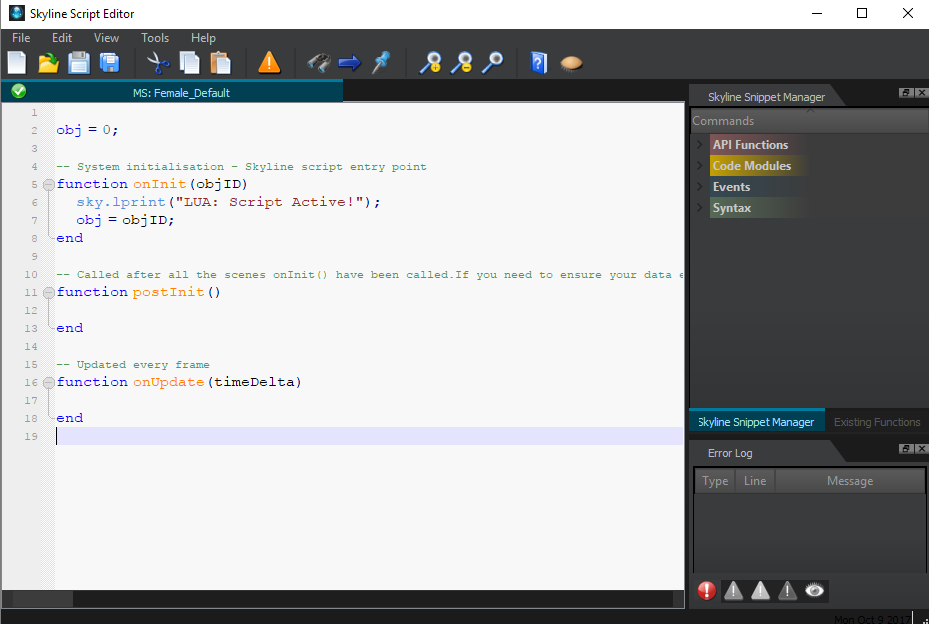
3a) Copy Paste Script
Select all of the script text and delete it, you should have a blank page. This is in preparation for adding the new movement example script.

3b) Save Script
Then all you need to do is press the save button. Clicking save in the script editor is context aware, i.e. it saves to the selected pages object if it is a micro script, or to a file location if it is an external script.

As we have mentioned Micro scripts are saved to the object not to file this means it is saved with the scene file, so make sure you save your scene regularly.
5) The Movement Script
Copy the following code and paste into the characters micro script. Then press the script editors save button and also save your scene.
walkSpeed = 1000; runSpeed = 2000; turnSpeed = 150; jumpAmt = 0.2; obj = 0; forwardKeyDown = false; backKeyDown = false; leftKeyDown = false; rightKeyDown = false; shiftKeyDown = false; spaceKeyDown = false; isJumping = false; idleanim = ""; walkanim = ""; runanim = ""; jumpanim = ""; timeDelta = 0.0; -- System initialisation - Skyline script entry point function onInit(objID) sky.lprint("LUA: Script Active!"); obj = objID; end -- Called after all the scenes onInit() have been called. -- If you need to ensure your data exits, then do you set up here function postInit() idleanim = anim.getFromMap(obj, "Idle1"); walkanim = anim.getFromMap(obj, "Walk"); runanim = anim.getFromMap(obj, "Run"); jumpanim = anim.getFromMap(obj, "Jump"); anim.playAnimation(obj, idleanim, 0, 0); end -- Updated every frame function onUpdate(td) timeDelta = td; checkForKeys() end function idle() character.move(obj, 0 ); anim.playAnimation(obj, idleanim,30, 1); end function walk(direction) character.move(obj, walkSpeed*direction*timeDelta ); anim.setSpeed(obj, walkanim, direction); anim.playAnimation(obj, walkanim, 30, 1); end function run(direction) character.move(obj, runSpeed*direction*timeDelta ); anim.setSpeed(obj, runanim, direction); anim.playAnimation(obj, runanim, 100, 1); end function turn( direction ) if(direction == -1)then entity.turn(obj, 0, turnSpeed*timeDelta, 0) elseif(direction == 1)then entity.turn(obj, 0, -turnSpeed*timeDelta, 0) end end function startJump() character.setJumpDownforce(obj, 1000); character.doJump(obj, 400 ); anim.playAnimation(obj, jumpanim, 10, 1); end function checkForKeys(timeDelta) if(forwardKeyDown == true and shiftKeyDown == false)then walk(1); elseif(forwardKeyDown == true and shiftKeyDown == true)then run(1); elseif(backKeyDown == true and shiftKeyDown == false)then walk(-1); elseif(backKeyDown == true and shiftKeyDown == true)then run(-1); else idle(); end if(spaceKeyDown == true)then startJump(); end if(leftKeyDown == true)then turn(-1, timeDelta); end if(rightKeyDown == true)then turn(1, timeDelta); end end function onKeyDown(key) if(key == "w")then forwardKeyDown = true; end if(key == "s")then backKeyDown = true; end if(key == "a")then leftKeyDown = true; end if(key == "d")then rightKeyDown = true; end if(key == "space")then spaceKeyDown = true; end if(key == "shift")then shiftKeyDown = true; end end function onKeyUp( key ) if(key == "w")then forwardKeyDown = false; end if(key == "s")then backKeyDown = false; end if(key == "a")then leftKeyDown = false; end if(key == "d")then rightKeyDown = false; end if(key == "space")then spaceKeyDown = false; end if(key == "shift")then shiftKeyDown = false; end end
If you would like to know more about how this script works please read to the end of the next section, as after the walking and running speed has been covered you will be able to skip the page if you are not interested in the scripting!